Yvan Major
Member
Hi everyone, i am trying to make the OSI buyable BOD Covers Token.
So far i got it to work but when i select a choice the bag doesn't drop.
If anyone is able to help that would be awesome. Thanks a lot!
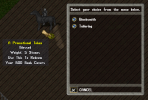
So far i got it to work but when i select a choice the bag doesn't drop.
If anyone is able to help that would be awesome. Thanks a lot!
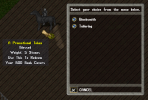
Code:
using System;
using Server;
using Server.Network;
using Server.Engines.VeteranRewards;
using Server.Gumps;
namespace Server.Items
{
public enum CoverBagType
{
Blacksmith = 100,
Tailoring = 101
}
public class BODBookCoverBag : Bag, IRewardItem
{
private bool m_IsRewardItem;
[Constructable]
public BODBookCoverBag(CoverBagType type)
: base()
{
switch ( type )
{
case CoverBagType.Blacksmith:
this.AddItem(new AgapiteBulkOrderCover());
this.AddItem(new BronzeBulkOrderCover());
break;
case CoverBagType.Tailoring:
this.AddItem(new AgapiteBulkOrderCover());
this.AddItem(new BronzeBulkOrderCover());
break;
}
}
public BODBookCoverBag(Serial serial)
: base(serial)
{
}
[CommandProperty(AccessLevel.GameMaster)]
public bool IsRewardItem
{
get
{
return this.m_IsRewardItem;
}
set
{
this.m_IsRewardItem = value;
this.InvalidateProperties();
}
}
public override void Serialize(GenericWriter writer)
{
base.Serialize(writer);
writer.WriteEncodedInt(0); // version
writer.Write((bool)this.m_IsRewardItem);
}
public override void Deserialize(GenericReader reader)
{
base.Deserialize(reader);
int version = reader.ReadEncodedInt();
this.m_IsRewardItem = reader.ReadBool();
}
}
public class BODBookCoverToken : Item, IRewardItem, IRewardOption
{
private CoverBagType m_BagType;
private bool m_IsRewardItem;
[Constructable]
public BODBookCoverToken()
: base(0x2AAA)
{
this.LootType = LootType.Blessed;
}
public BODBookCoverToken(Serial serial)
: base(serial)
{
}
public override int LabelNumber
{
get
{
return 1070997;
}
}// A promotional token
[CommandProperty(AccessLevel.GameMaster)]
public bool IsRewardItem
{
get
{
return this.m_IsRewardItem;
}
set
{
this.m_IsRewardItem = value;
this.InvalidateProperties();
}
}
public override void OnDoubleClick(Mobile from)
{
if (this.m_IsRewardItem && !RewardSystem.CheckIsUsableBy(from, this, null))
return;
if (this.IsChildOf(from.Backpack))
{
from.CloseGump(typeof(RewardOptionGump));
from.SendGump(new RewardOptionGump(this));
}
else
from.SendLocalizedMessage(1062334); // This item must be in your backpack to be used.
}
public override void GetProperties(ObjectPropertyList list)
{
base.GetProperties(list);
list.Add(1070998,"BOD Book Covers"); // Use this to redeem<br>your ~1_PROMO~
if (this.m_IsRewardItem)
list.Add(1076218); // 2nd Year Veteran Reward
}
public override void Serialize(GenericWriter writer)
{
base.Serialize(writer);
writer.WriteEncodedInt(0); // version
writer.Write((bool)this.m_IsRewardItem);
}
public override void Deserialize(GenericReader reader)
{
base.Deserialize(reader);
int version = reader.ReadEncodedInt();
this.m_IsRewardItem = reader.ReadBool();
}
public void GetOptions(RewardOptionList list)
{
list.Add((int)CoverBagType.Blacksmith, 1002043); // Blacksmith
list.Add((int)CoverBagType.Tailoring, 1002155); // Tailoring
}
public void OnOptionSelected(Mobile from, int option)
{
this.m_BagType = (CoverBagType)option;
if (!this.Deleted)
base.OnDoubleClick(from);
}
}
}