Apparently so!
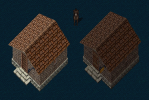
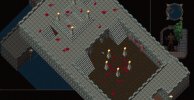
Issue: Requires UltimaSDK or OpenUO
So, now that we know it is possible, I will try to port some UOSDK/OpenUO code to allow this to be implemented without those respective assemblies and with any luck, be able to release a stand-alone version as well as including it in the next VNc update.
For now, I just wanted to spark some discussion on the possibilities of uses for this feature; at the moment, it supports any MultiComponentList (all BaseMultis have this, via the Components property) - so you can not only display any multi, but you can also display custom house designs...
The final release will simply use a tile matrix, something like StaticTile[][][], to allow you to build any kind of tile-based structure in a gump, as if they were drawn in the client; I foresee it possibly being used for a new kind of Addon Designer. However, the code is quite simplistic at the moment and would require significant interface boilerplate code to function anything like a designer - for now, it just displays the tile matrix.
A code sample, for those who want to know;
So, what's on your mind now?
[EDIT]
Addition of the UltimaArtExt class code that I forgot to post originally with the above example - this code is required if you wish to implement this feature right now on your shard, but you must have Ultima.dll installed and the Ultima namespace available.
This code is responsible for the proper placement of tiles in gumps, it can also be used in various other places, for example, properly aligning items displayed as icons, without having to hard-code the image's offset.
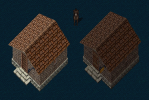
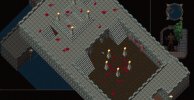
Issue: Requires UltimaSDK or OpenUO
So, now that we know it is possible, I will try to port some UOSDK/OpenUO code to allow this to be implemented without those respective assemblies and with any luck, be able to release a stand-alone version as well as including it in the next VNc update.
For now, I just wanted to spark some discussion on the possibilities of uses for this feature; at the moment, it supports any MultiComponentList (all BaseMultis have this, via the Components property) - so you can not only display any multi, but you can also display custom house designs...
The final release will simply use a tile matrix, something like StaticTile[][][], to allow you to build any kind of tile-based structure in a gump, as if they were drawn in the client; I foresee it possibly being used for a new kind of Addon Designer. However, the code is quite simplistic at the moment and would require significant interface boilerplate code to function anything like a designer - for now, it just displays the tile matrix.
A code sample, for those who want to know;
C#:
public class MultiDisplayGump : SuperGump
{
public static void Initialize()
{
CommandUtility.Register(
"ViewMulti",
AccessLevel.GameMaster,
e => e.Mobile.BeginTarget<Server.Multis.HouseSign>(
(m, s) =>
{
new MultiDisplayGump((PlayerMobile)m, s.House).Send();
},
null));
}
public BaseMulti Multi { get; set; }
public MultiDisplayGump(PlayerMobile user, BaseMulti multi)
: base(user)
{
Multi = multi;
Modal = true;
}
protected override void Compile()
{
base.Compile();
CanMove = true;
}
protected override void CompileLayout(SuperGumpLayout layout)
{
base.CompileLayout(layout);
layout.Add("multi", () => AddMulti(0, 0, Multi, Multi.Hue));
}
public virtual void AddMulti(int x, int y, int multiID, int hue)
{
AddMulti(x, y, MultiExtUtility.GetComponents(multiID), hue);
}
public virtual void AddMulti(int x, int y, BaseMulti multi, int hue)
{
AddMulti(x, y, multi.Components, hue);
}
public virtual void AddMulti(int x, int y, MultiComponentList mcl, int hue)
{
x += 22 * mcl.Height;
Point offset;
int h;
for (var xo = 0; xo < mcl.Width; xo++)
{
for (var yo = 0; yo < mcl.Height; yo++)
{
foreach (var t in mcl.Tiles[xo][yo].Where(t => t.ID > 1 && t.ID <= TileData.MaxItemValue)
.OrderBy(zt => zt.Z)
.ThenByDescending(zt => TileData.ItemTable[zt.ID].Flags.HasFlag(TileFlag.Surface))
.ThenByDescending(zt => TileData.ItemTable[zt.ID].Flags.HasFlag(TileFlag.Wall))
.ThenBy(zt => TileData.ItemTable[zt.ID].Flags.HasFlag(TileFlag.Roof))
.ThenBy(zt => TileData.ItemTable[zt.ID].CalcHeight))
{
offset = UltimaArtExt.GetImageOffset(t.ID);
offset = new Point(((xo * 22) - (yo * 22)) + offset.X, (((yo * 22) + (xo * 22)) + offset.Y) - (t.Z * 4));
h = t.Hue > 0 ? t.Hue : hue;
if (h > 0)
{
AddItem(x + offset.X, y + offset.Y, t.ID, h);
}
else
{
AddItem(x + offset.X, y + offset.Y, t.ID);
}
}
}
}
}
}
So, what's on your mind now?
[EDIT]
Addition of the UltimaArtExt class code that I forgot to post originally with the above example - this code is required if you wish to implement this feature right now on your shard, but you must have Ultima.dll installed and the Ultima namespace available.
This code is responsible for the proper placement of tiles in gumps, it can also be used in various other places, for example, properly aligning items displayed as icons, without having to hard-code the image's offset.
C#:
#region Header
// Voxpire _,-'/-'/ ArtExt.cs
// . __,-; ,'( '/
// \. `-.__`-._`:_,-._ _ , . ``
// `:-._,------' ` _,`--` -: `_ , ` ,' :
// `---..__,,--' (C) 2015 ` -'. -'
// # Vita-Nex [http://core.vita-nex.com] #
// {o)xxx|===============- # -===============|xxx(o}
// # The MIT License (MIT) #
#endregion
#region References
using System.Drawing;
using Server.Items;
using Ultima;
#endregion
namespace Server
{
public static class UltimaArtExt
{
public static Point GetImageOffset(int id)
{
var b = GetImageSize(id);
int x = 0, y = 0;
if (b.Width > 44)
{
x -= (b.Width - 44) / 2;
}
else if (b.Width < 44)
{
x += (44 - b.Width) / 2;
}
if (b.Height > 44)
{
y -= (b.Height - 44);
}
else if (b.Height < 44)
{
y += (44 - b.Height);
}
return new Point(x, y);
}
public static Size GetImageSize(int id)
{
var img = id >= 0 && id <= TileData.MaxItemValue ? Art.GetStatic(id) : null;
if (img == null)
{
return new Size(44, 44);
}
return new Size(img.Width, img.Height);
}
public static Size GetImageSize(this Item item)
{
if (item == null || item is BaseMulti)
{
return new Size(44, 44);
}
return GetImageSize(item.ItemID);
}
public static Rectangle2D GetStaticBounds(int id)
{
var img = id >= 0 && id <= TileData.MaxItemValue ? Art.GetStatic(id) : null;
if (img == null)
{
return new Rectangle2D(0, 0, 44, 44);
}
int xMin, xMax, yMin, yMax;
Art.Measure(img, out xMin, out yMin, out xMax, out yMax);
return new Rectangle2D(new Point2D(xMin, yMin), new Point2D(xMax, yMax));
}
public static Rectangle2D GetStaticBounds(this Item item)
{
if (item == null || item is BaseMulti)
{
return new Rectangle2D(0, 0, 44, 44);
}
return GetStaticBounds(item.ItemID);
}
}
}
Last edited: